WCTL
Server-side Scripting
WCTL
Loops
Introduction
Split
Break
Continue
Troubleshooting
Resources
Next Section
Introduction
In the
previous section, you learned
about conditionals - how to test for different conditions and
take the appropriate action depending on the results.
In this
section you will learn a powerful tool that allows you to automate
repetitive tasks - a programming feature called looping.
To get
started, place the following WCTL in your test folder header (go
back to the intro section if you
need help in doing this):
%% set number 1 %%
%% while number <=10 %%
%% number %% <BR>
%% set number number + 1 %%
%% endwhile %%
Look
at the result of executing this WCTL code by entering the test
folder. You should see the numbers 1 through 10, each displayed
on a separate line.
The while
keyword marks the beginning of a loop section. The endwhile
keyword marks the end of the section.
Let's
go through this WCTL line-by-line to make sure we understand it
all:
- %%
set number 1 %%
number
is just the name of a variable. We store the value 1
in number because the first value we want to show is 1.
(Programmers would say we have "initialized" number with the
value 1.)
- %%
while number <=10 %%
The
format of a while statement is
%% while condition %%
As long as the condition (which we studied in the previous
section) is true, everything in the while section is repeated
over and over again. In this example, so long as the value of
number is less than or equal to 10, the while loop will continue
to execute.
- %%
number %% <BR>
The
value of the variable number is output to the web page,
followed by a <BR> tag - an HTML line break. If you left
out the <BR> tag, all 10 numbers would run together on
the same line.
- %%
set number number + 1 %%
Here
we increment number (add 1 to number).
If we left out this important line the value of number would
never change and the condition in the while statement (number
<=10) would always be true!
- %%
endwhile %%
This marks the end of the while loop.
Note:
So, what would actually happen if you left out the %%
set number number + 1 %% line? Would the while loop
really never stop? Those of you who are courageous enough
to try this, go ahead. Remove that line from your WCTL and
see what happens.
A
lot of lines with 1 are shown. An awful lot of lines.
Hundreds in fact. But the while loop did, in fact, stop.
<whew!>
To
prevent mistakes like that from occurring (a situation known
by programmers as an "infinite loop") Web Crossing limits
the number of times a while loop can execute. You can increase
or decrease the default value for this limit in Control
Panel > General Settings > Maximum while-loop iterations
per page:.
If you are ever running a while loop and you feel that the
loop is ending sooner than you expect and you cannot find
any other reason for it, check that setting. It may be too
low. One common situation where this might occur is if you
have a large number of users and you want to loop through
all your users.
|
In plain
English, you would read this test WCTL as follows:
- First
set number to be 1.
- While
number is less than or equal to 10, output number
and then increment number.
The concept
is easy to understand. But why would you want to loop in webx?
Well,
for example, lets say you wanted to generate a list of all the
users and their email addresses. Using a while loop you could
show one user and his or her email address on a line, or inside
a nice-looking table.
Or suppose
you didn't like the standard list of items in a folder, and you
wanted to make your own list, formatted in a completely different
way, perhaps using a multiple-column table. You could use a while
loop and loop through all the items inside a folder, showing the
items however you want.
There
are really an unlimited number of situations for using while loops
inside Web Crossing. And there are a few commands to help make
things easier.
Using
the split Command
Let's
try a looping experiment in a folder to make all this clearer.
First, create a test folder called Pets. Add as many discussions
as you like to the folder. Figure 1 shows what our Pets
folder looks like (yours can be different):
Figure
1 - A Test Pets Folder
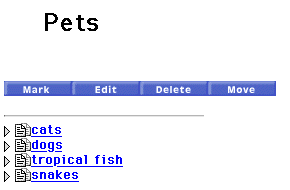
For an
experiment, lets use the names of all the discussions contained
inside Pets to automatically create an interesting folder
header. We can do this with the following WCTL code. Place the
code inside the header and see for yourself how it works:
All about:
%% set items pathSelect %%
%% set item items.split %%
%% while item %%
%% setPath(item) %%
%% pathTitle %% and
%% set item items.split %%
%% endwhile %%
other pets...
In our
case, when we enter the folder, the folder header now says:
- All
about: cats and dogs and tropical fish and snakes and other
pets...
Try it
with your folder and see the results. Try adding more discussions
and see how the folder header automatically add the names of the
new discussions to the folder header.
Well,
this example uses a number of concepts that are new to you, so
lets examine this code line-by-line to make sure it is all clear:
- All
about:
This line is just ordinary text (it isn't between %% marks),
so that text is just displayed as is.
- %%
Set items pathSelect %%
pathSelect
is a WCTL built-in variable whose value is a blank-delimited
character string containing all the unique ID numbers for all
the items at the present location.
For example, if you have three items (discussions) in your Pets
folder pathSelect would have a value that looked something
like ".ee6d6d6 .ee6d6d7 .ee6d6d8", though the actual numbers
would be different for your Web Crossing site.
What this line does is initialize a local variable called items
with the list of discussion IDs in the Pets folder. We
can then use items in our while loop.
For a complete list of WCTL built-in variables, see the Web
Crossing Sysop Docs web page.
- %%
Set item items.split %%
You
can add .split to the end of any variable. What Split
does is split off the first word (words are separated by blanks)
contained in the variable and leave the rest of the character
string inside untouched.
For example, if the variable items contains the character
string "today it is sunny" then the value of items.split
is "today" and after the split items will contain
the remaining string "it is sunny".
So what we are doing here is just setting the variable item
to the first word in items - the unique ID number for
the first discussion in the folder.
- %%
while item %%
So
long as item contains a value the loop will repeat itself.
- %%
setPath(item) %%
Web
Crossing is very conscious of "where you are" at any time and
calls this location a path. Many WCTL commands use the
current path when performing basic operations like displaying
a title or author.
For example, the WCTL command %% pathTitle %% shows the
title of whatever the current path is - the current folder,
discussion, message, chat room or link name.
setPath is a WCTL command that sets your current location
temporarily to the location of the folder, discussion, message
or chat room that you specify inside the parentheses.
Here we are setting the path to item so that we can then
use the pathTitle command to show the title of the discussion
specified by the discussion number stored in item.
- %%
pathTitle %% and
Here
we are just displaying the current path title (the title of
the current discussion in our loop). The word and is
outside %% marks, so it is treated as ordinary HTML -
just a word to be output to the folder header.
- %%
Set item items.split %%
At
the end of the loop section we split off the next discussion
ID number and store it in item. If there are no more discussion
numbers in items, then a null value (an empty character string)
is stored in item. That is how the while loop ends! The while
loop is always testing to see if item contains a value. When
it no longer contains a value, the while loop test condition
is no longer true and the loop stops.
- %%
endwhile %%
The
endwhile keyword marks the end of a while section.
- other
pets...
This
is just ordinary HTML - extra words to add to the folder header
after the while loop is done.
Well,
this section threw quite a few new concepts at you - while loops,
paths and the split command. Try this WCTL and variations of it
in a test folder to get a better feel for how it works.
The
break Command
Sometimes
you want to finish up a while loop even before the test condition
becomes false. For example, you might find some unexpected result
in the middle of your loop and decide not to continue. You can
use the break command to get out of a loop immediately.
For example, consider the following WCTL code (that you can also
try out in your test folder header):
%% set users selectUsers %%
%% set thisUser users.split %%
%% while thisUser %%
%% if thisUser.userName == "Hacker
King" %%
Warning! Hacker King should
not be a member!
%% break %%
%% endif %%
%% thisUser.userName %% is a member in good standing
<BR>
%% set thisUser users.split %%
%% endwhile %%
Here
we are using split and while to loop to go through a list of users
one-by-one, displaying a message saying that the user is in good
standing. However if for some reason one of the user's names is
"Hacker King" we realize something is wrong and we break out of
the loop without finishing.
The selectUsers
command returns a list of users. (There are lots of options that
can be used with the selectUsers command. You can read about them
all in the sysop
reference documents section on selectUsers. Without any options,
as we have used it here, selectUsers just returns the first 50
users in your database.)
The
continue Command
Unlike
the break command, where you want to quit the while loop
entirely, the continue command gives you the ability to
stop executing from the middle of a while loop and continuing
from the start of the loop again.
For example,
consider the following WCTL code that divides 10 by all the numbers
from -5 to 5:
%% set n -6 %%
%% while n < 5 %%
%% set n n + 1 %%
%% if n == 0 %%
%% continue %%
%% endif %%
10 divided by %% n %% is %% 10 / n %% <BR>
%% endwhile %%
Try this
in your test folder. You will see that a line is output for each
calculation and the case where n is equal to zero is neatly avoided
with the use of the %% continue %% statement.
By the
way, all the results are truncated to integer (round) values;
WCTL only does integer arithmetic. If you need to do more sophisticated
numerical calculations you should use Web Crossing's Server-side
JavaScript.
The next
section describes you can create your own commands (macros)
which can be used from anywhere inside Web Crossing - not just
in your test folder.
Troubleshooting
I
tried the examples above, but when I look at the header all I
see is the WCTL itself, with all the double-percent marks. What
am I doing wrong?
- Make
sure you have turned on WCTL evaluation in the Control
Panels > General Settings:

Resources
Web Crossing
FAQ:
Sysop
docs:
WCTL
Concept Reference Page
Web
Crossing Tech Support Forum
Developer
Center
WebX
Harbor
|